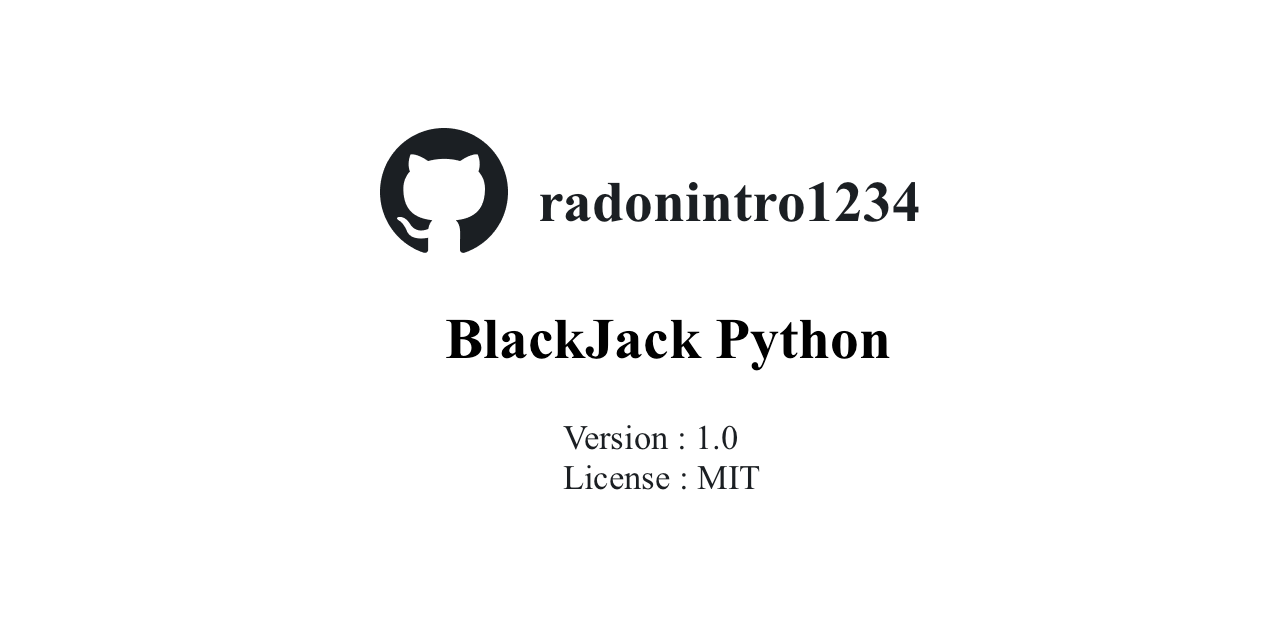
Projects > C++ Blackjack ExampleI created a simple blackjack example using C++ that allows the player to play against a CPU opponent and whoever gets closest to 21 without going over wins. This is a simple blackjack example because players only have the option to hit or stay each turn. //Specification: This program plays a version of //the card game of 21. //A human player is pitted against the computer. //The player who is the closest to 21 without //going over wins the hand. #include <iostream>#include <ctime>#include <string>using namespace std;//prototypes... void play21(void);int dealCards(int, string);void hit(int &);void determineWinner(int, int);int Random(int, int);void main(){char keepPlaying = 'n'; //loop control variable do {play21(); //keep playing?cout << 'Do you want to play another hand (y/n)?';cin >> keepPlaying; } while(keepPlaying 'Y' keepPlaying 'y');} void play21(void){//play one hand of 21//randomize the cardssrand(( int) time(0));// deal the cardsint person = dealCards(2, 'Your Cards:');cout << ' = ' << person << endl;int house = dealCards(2, 'Computers Cards:');cout << ' = ' << house << endl;// Ask if human wants a hithit(person); cout << endl; //Determine if computer takes a hitwhile ((house < person) && (house <= 21) && (person <= 21)) {house += dealCards(1, 'The Computer takes a card ');cout << endl; } //show who won....determineWinner(person, house); } void determineWinner(int humanScore, int houseScore) {//compare the scores to see who won//Both the human and the house score totals are provided as arguments//Display total scores and indicate winner//possible outcomes: human wins, computer wins, tie//display the scorescout << 'Your Score: ' << humanScore << endl;cout << 'Computer Score: ' << houseScore << endl;//decide who should winif (humanScore houseScore) cout << 'Tie';if ((humanScore 21 humanScore >= houseScore houseScore > 21) && (humanScore <= 21))cout << 'nYou Won!n';elsecout << 'nThe Computer won!n';} int dealCards(int numberOfCards, string message){//deals cards//The number of cards to be delt is provided as an argument//A message indicating which player is recieving the cards is also//given as an argument //The player message and the cards delt is displayed to the screen//the total value of the cards delt is returnedint cardDealt = 0;int totalValue = 0;cout << message << ' ';//deal the number of required cardsfor (int i = 1 ; i <= numberOfCards ; i++){//get a cardcardDealt = Random(1, 10); //accumulate the card valuestotalValue += cardDealt; cout << cardDealt << ' ';} return totalValue;} void hit(int &playerScore){//Ask the human if they want another card -- 'a hit'//the player's score total is accumulated as they take cards//the player can contiue taking cards until they wish to stop or they exceed 21//After a card is taken the user's current total is displayed//If the user goes over 21 'busted' is displayed//Keep giving the player cards until he wants to stop or goes over 21char takeHit = 'Y';while (takeHit != 'n') {if (playerScore < 21) {//does the player want a hit?cout << 'do you want a hit (Y/N)? ';cin >> takeHit; if (takeHit 'Y' takeHit 'y'){//the player wants a hit so deal a cardplayerScore += dealCards(1, 'Hit: ');cout << 'Your total is ' << playerScore << endl;} else//the player does not want another cardtakeHit = 'n';} else {//the player has bustedif (playerScore > 21)cout << 'You Busted..nn';takeHit = 'n';} } } int Random(int lowerLimit, int upperLimit) {//returns a random number within the given boundary return 1 + rand() % (upperLimit - lowerLimit + 1);} |
|
Blackjack In C


C Language Blackjack Games
Count on a fun online game of Blackjack. Play for free online! Search MSN Games. Genre: Card & Casino. If you like Blackjack, you'll love Microsoft Bubble! Beat the dealer! Make your bet, and you're dealt two cards. If you think you can get closer to 21 without going over, have the dealer give you another. Simplified Blackjack in C 20 Dec 2010. Now I’d like to share one of my experience of programming C. Our teacher gave us a task: to finish a small but not so useless program by our own in C.